How to Send SMS Messages With PHP - A Step by Step Guide
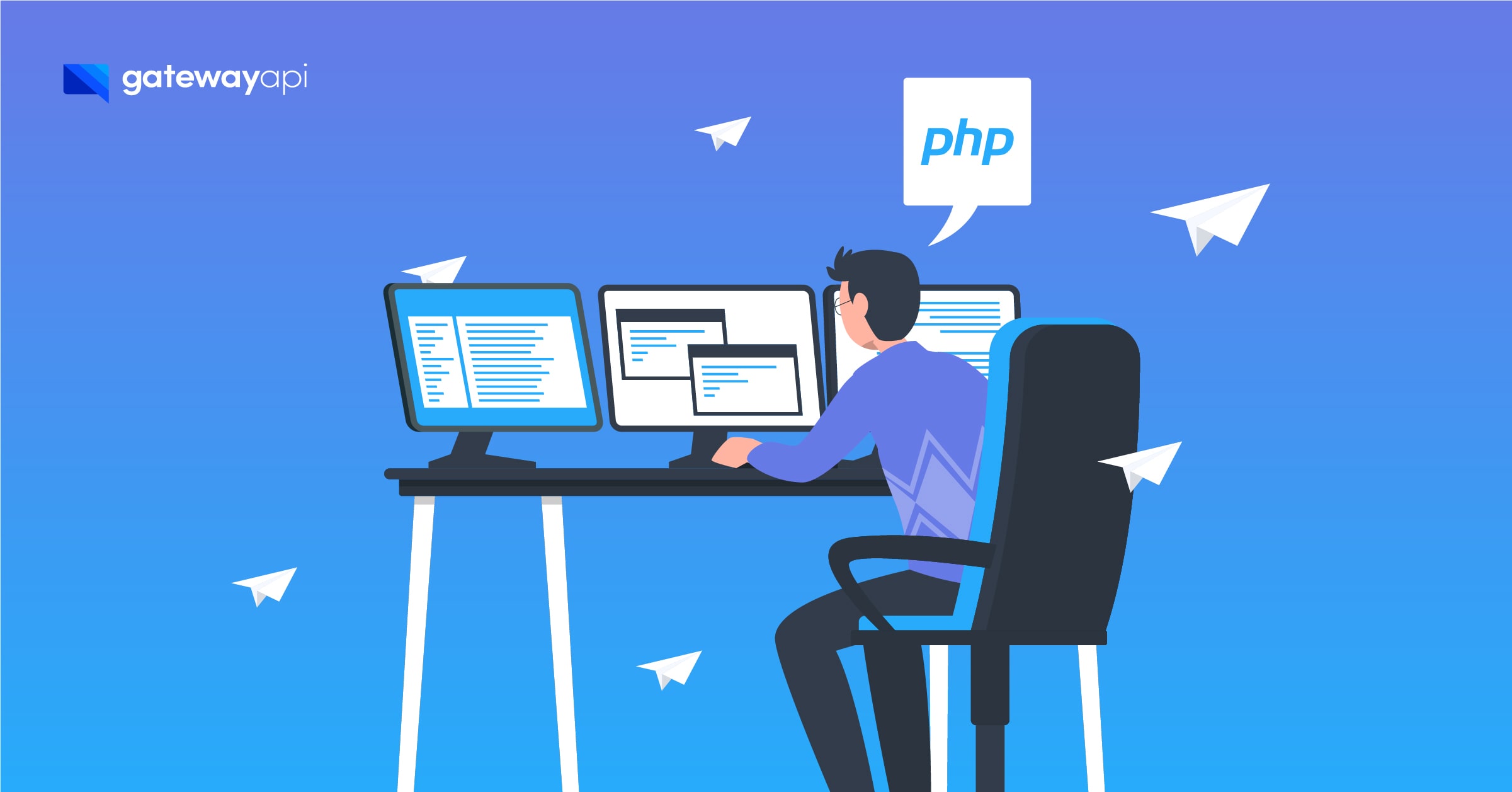
In this blog post, I will show you how you can send SMS messages using PHP.
Before we begin, it’s important that you have a basic understanding of PHP and a GatewayAPI account with account credit.
Now let’s get started with the actual sending. Once you have created an account and have access to your dashboard, you can see the code samples on the front page of your dashboard. Select the PHP example, copy and paste it into your favorite editor. Personally, I prefer Visual Studio Code, but you can use any editor you prefer.
After you insert the code, it will look something like this:
Step-by-step code walkthrough
In this section, I will walk you through each step of the code so you understand what it does and how you can customize it to your specific needs. If this seems straightforward, you can skip to the section where I execute the script.
In the first line, we start our PHP file. In the third line, we have a variable that contains the URL to our endpoint for sending SMS messages. Do not change this variable. In the fourth line we have your API token, which is used to identify you and your account.
In the code, we also have details about the content of the message. We have “recipients”, which are the phone numbers the message should be sent to. In the example we use a couple of test numbers, but you can insert up to 10,000 different recipients here.
First, enter your country code without any zeros or plus signs in front of it. For example, I use “45” for Denmark. Then you insert your own number.
In the next line we have a JSON object, which is the object that sends information to our server about how the SMS should be sent. First we have “sender”. This is the Sender ID that will be displayed on the recipient’s phone. For example, if you receive an SMS from your bank, the bank’s name will often be the sender. In the example, it automatically says “ExampleSMS”, but you can enter your own name or the desired Sender ID.
“Message” is of course the content of the SMS. In the example, I just write “Hello world”. Note that if you want to include emojis or special characters, it requires some extra steps, which you can find more information about in our documentation.
In the next line, we leave “recipients” blank because we fill it in the following line, where we use a for loop that takes each recipient and adds them to our recipient array.
cURL
Now our JSON object is ready to be sent to the server. To do this, we use the cURL library built into PHP. We start by initializing our cURL object using “curl_init()” and store it in the variable “$ch”. Then we set up some parameters for our cURL object using “curl_setopt()”. We specify the URL where the request should be sent to, set the “Content-Type” to “application/JSON” to tell the server that we are sending a JSON object, and use our API token as the username and no password.
After setting up the cURL object, we are ready to execute the request itself by calling “curl_exec($ch)”. This will send the HTTPS request with the specified parameters and store the response from the server in the “$result” variable. Then we close the cURL object with “curl_close($ch)”.
To see the response from the server, we print “$result” using “print($result)”. If everything goes as planned, you will see the server’s response in your output.
Furthermore, we decode the JSON object to access specific information using “json_decode($result, true)”. In the example, we print IDs from the JSON object using “print_r($response[‘ids’])”. This ID can be used as a reference if you need support or similar.
Executing the PHP script
Our PHP script is ready and we can now execute it. You can run the script any way you like. I prefer to use my terminal on my computer.
First, navigate to the folder where your file is located and then you can run the script by typing “php sms.php” in the terminal.
Once the script has been executed, you should receive an SMS on your phone.

Screenshot from my terminal where the script is running
The response you receive contains some important information. First of all, IDs are your “message ID”, which is the identification of the message you just sent. This ID can be used in our system and you can also use it as a reference if you need support or want to track the message.
“Usage” indicates the number of messages you have sent and to which countries. For example, “DK: 1” means that one message was sent to Denmark. “Currency” shows the currency used and the price shows the specific cost of the message.
Concluding remarks
As a final note, I’d like to share a few things to be aware of when sending SMS messages via an SMS API, like the ones we offer.
Firstly, the “sender” field has some limitations. Avoid using special characters or symbols as this can result in the message not being sent correctly or the Sender ID being overwritten.
There is also a limit of 11 characters (alphanumerical), or 15 numbers (numerical), for Sender IDs, so make sure to keep it at or below this length.
If you want to include links in your messages, you must first go through the whitelisting process on your GatewayAPI profile. It usually takes no more than one business day to get a link approved, but make sure to do it well in advance of the planned sending.
One last thing to be aware of is to make sure that the encoding of your SMS or PHP file is set to UTF-8. This is usually the default setting in most systems, but for Windows users, there may be situations where the PHP script is set to a different encoding. It’s important to make sure both are in the same encoding to avoid problems.
What I’ve shown you today are just the basic steps to send an SMS message. GatewayAPI has much more to offer and you can read more about it in our documentation.
You can also visit our YouTube channel where my colleague walks you through how to send messages via PHP in exactly the same way as in this blog post.
In addition, you can find a complete PHP library for integration with GatewayAPI on GitHub.
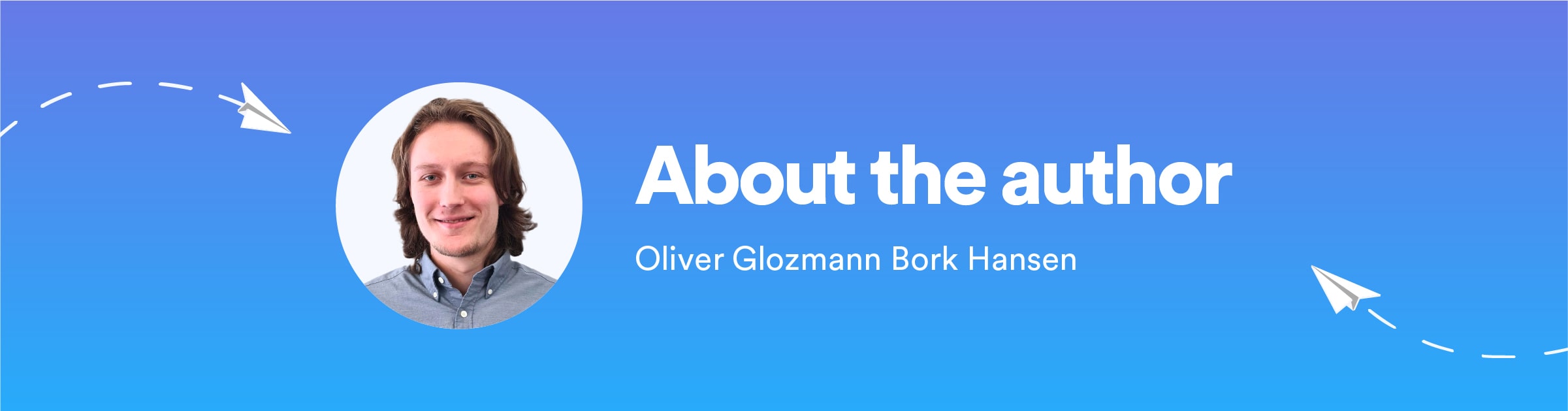
About the author
Oliver focuses on integrations and is the man to go to if you need an integration with your preferred system. When he is not working on GatewayAPI, he is an avid student of AI and Deep Learning. Oliver generally just enjoys working with code, whether it’s AI or something more down-to-earth, such as GUI or backend software.